Problem
You are given an integer array height
of length n
. There are n
vertical lines drawn such that the two endpoints of the ith
line are (i, 0)
and (i, height[i])
.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return the maximum amount of water a container can store.
Notice that you may not slant the container.
Example 1:
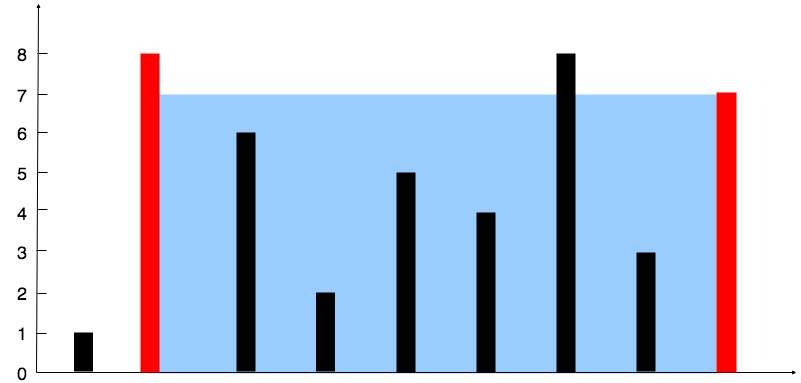
Input: height = [1,8,6,2,5,4,8,3,7] Output: 49 Explanation: The above vertical lines are represented by array [1,8,6,2,5,4,8,3,7]. In this case, the max area of water (blue section) the container can contain is 49.
Example 2:
Input: height = [1,1] Output: 1
Solution
Bruteforce solution with O(n2) time complexity
// brute force solution O(n2) time complexity
var maxArea = function(height) {
let biggestarea = 0;
for(let i = 0; i<height.length; i++){
for(let j = i+1; j<height.length;j++){
let smallerheight = (height[i] > height[j])?height[j]:height[i];
let area = Math.abs(smallerheight)*Math.abs(i-j);
if(area > biggestarea){
biggestarea = area;
}
}
}
return biggestarea;
};
Optimal solution with O(n) complexity
// optimal solution with O(n) time complexity
var maxArea = function(height) {
let biggestarea = 0;
let left = 0; let right = height.length -1;
while(left < right){
let smallerheight = (height[left] > height[right])?height[right]:height[left];
let area = Math.abs(smallerheight)*Math.abs(left-right);
if(area > biggestarea){
biggestarea = area;
}
if(height[left] < height[right]){
left++;
}
else{
right--;
}
}
return biggestarea;
};
A freelance web developer with a decade of experience in creating high-quality, scalable web solutions. His expertise spans PHP, WordPress, Node.js, MySQL, MongoDB, and e-commerce development, ensuring a versatile approach to each project. Aadi’s commitment to client satisfaction is evident in his track record of over 200 successful projects, marked by innovation, efficiency, and a customer-centric philosophy.
As a professional who values collaboration and open communication, Aadi has built a reputation for delivering projects that exceed expectations while adhering to time and budget constraints. His proactive and problem-solving mindset makes him an ideal partner for anyone looking to navigate the digital landscape with a reliable and skilled developer.