Problem
You are given two strings, coordinate1
and coordinate2
, representing the coordinates of a square on an 8 x 8
chessboard.
Below is the chessboard for reference.
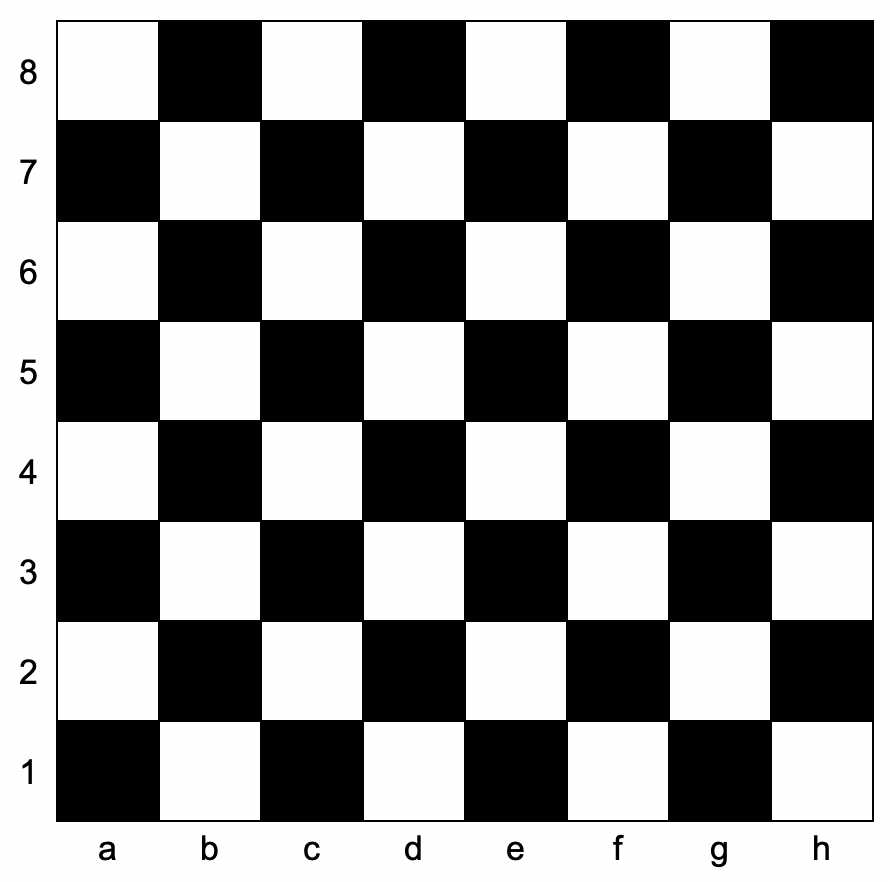
Return true
if these two squares have the same color and false
otherwise.
The coordinate will always represent a valid chessboard square. The coordinate will always have the letter first (indicating its column), and the number second (indicating its row).
Example 1:
Input: coordinate1 = “a1”, coordinate2 = “c3”
Output: true
Explanation:
Both squares are black.
Example 2:
Input: coordinate1 = “a1”, coordinate2 = “h3”
Output: false
Explanation:
Square "a1"
is black and "h3"
is white.
Constraints:
coordinate1.length == coordinate2.length == 2
'a' <= coordinate1[0], coordinate2[0] <= 'h'
'1' <= coordinate1[1], coordinate2[1] <= '8'
Solution
First attempt : Time complexity O(n2)
impl Solution {
pub fn check_two_chessboards(coordinate1: String, coordinate2: String) -> bool {
let mut color1 : &str = "";
let mut color2 : &str = "";
let mut output : bool = true;
// coordinate 1
let mut coor1array : Vec<&str> = coordinate1.split("").filter(|&s| !s.is_empty()).collect();
if let Ok(num1) = coor1array[1].parse::<i32>() {
if((coor1array[0] == "a" || coor1array[0] == "c" || coor1array[0] == "e" || coor1array[0] == "g") && num1 % 2 == 0 ){
color1 = "White";
}
else if((coor1array[0] == "a" || coor1array[0] == "c" || coor1array[0] == "e" || coor1array[0] == "g") && num1 % 2 != 0 ){
color1 = "Black";
}
else if((coor1array[0] == "b" || coor1array[0] == "d" || coor1array[0] == "f" || coor1array[0] == "h") && num1 % 2 == 0 ){
color1 = "Black";
}
else if((coor1array[0] == "b" || coor1array[0] == "d" || coor1array[0] == "f" || coor1array[0] == "h") && num1 % 2 != 0 ){
color1 = "White";
}
}
// coordinate 2
let mut coor2array : Vec<&str> = coordinate2.split("").filter(|&s| !s.is_empty()).collect();
if let Ok(num2) = coor2array[1].parse::<i32>() {
if((coor2array[0] == "a" || coor2array[0] == "c" || coor2array[0] == "e" || coor2array[0] == "g") && num2 % 2 == 0 ){
color2 = "White";
}
else if((coor2array[0] == "a" || coor2array[0] == "c" || coor2array[0] == "e" || coor2array[0] == "g") && num2 % 2 != 0 ){
color2 = "Black";
}
else if((coor2array[0] == "b" || coor2array[0] == "d" || coor2array[0] == "f" || coor2array[0] == "h") && num2 % 2 == 0 ){
color2 = "Black";
}
else if((coor2array[0] == "b" || coor2array[0] == "d" || coor2array[0] == "f" || coor2array[0] == "h") && num2 % 2 != 0 ){
color2 = "White";
}
}
if(color2 == color1){
output = true;
}
else{
output = false;
}
return output
}
}
A freelance web developer with a decade of experience in creating high-quality, scalable web solutions. His expertise spans PHP, WordPress, Node.js, MySQL, MongoDB, and e-commerce development, ensuring a versatile approach to each project. Aadi’s commitment to client satisfaction is evident in his track record of over 200 successful projects, marked by innovation, efficiency, and a customer-centric philosophy.
As a professional who values collaboration and open communication, Aadi has built a reputation for delivering projects that exceed expectations while adhering to time and budget constraints. His proactive and problem-solving mindset makes him an ideal partner for anyone looking to navigate the digital landscape with a reliable and skilled developer.
Hello.
Good cheer to all on this beautiful day!!!!!
Good luck 🙂