Problem
Given the root
of a binary tree, return the preorder traversal of its nodes’ values.
Example 1:
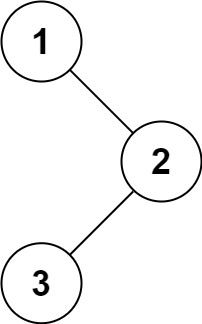
Input: root = [1,null,2,3] Output: [1,2,3]
Solution
/**
* Definition for a binary tree node.
* function TreeNode(val, left, right) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
*/
/**
* @param {TreeNode} root
* @return {number[]}
*/
var preorderTraversal = function(root) {
let result = new Array();
if(root){
if(root.left == null && root.right == null){
result.push(root.val);
}
else if(root.left != null || root.right != null){
result = preordertraverse(root, result);
}
}
return result;
};
function preordertraverse(root, arr){
if(!root){
return null;
}
else{
arr.push(root.val);
preordertraverse(root.left, arr);
preordertraverse(root.right, arr);
}
return arr;
}
A freelance web developer with a decade of experience in creating high-quality, scalable web solutions. His expertise spans PHP, WordPress, Node.js, MySQL, MongoDB, and e-commerce development, ensuring a versatile approach to each project. Aadi’s commitment to client satisfaction is evident in his track record of over 200 successful projects, marked by innovation, efficiency, and a customer-centric philosophy.
As a professional who values collaboration and open communication, Aadi has built a reputation for delivering projects that exceed expectations while adhering to time and budget constraints. His proactive and problem-solving mindset makes him an ideal partner for anyone looking to navigate the digital landscape with a reliable and skilled developer.