What are prototypes in JavaScript?
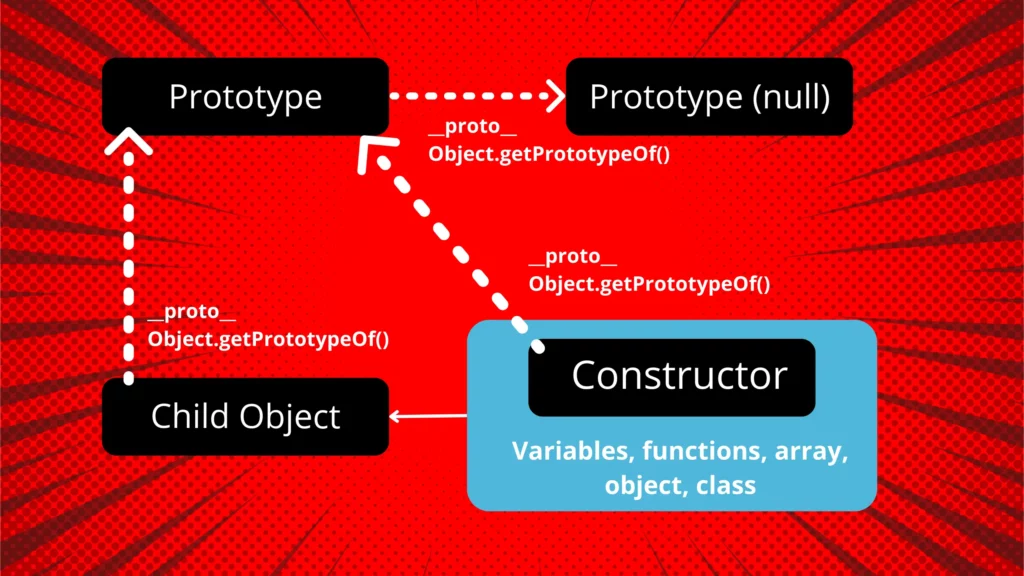
Whenever we create objects, functions, methods, arrays and variables in JavaScript, prototype is an object that gets attached to them. Prototype has some hidden properties which we can use with whatever prototype object is attached to.
I have always wondered that where do all the methods comes from in JavaScript objects, for example, if we initialize an array like below :
let anarray = ['1','2','3'];
There are some many thins we can do with this anarray
. Like below:
anarray.length; // this will give length of this array
So where does this length functionality comes from?
The answer is prototypes.
As we all know, everything in JavaScript is an Object and even if we create a string, Array etc then we are just copying the Object from the predefined objects in JavaScript like String, Array respectively. Every object in javascript has a constructor function, that constructor function has a prototype method attached to it, this prototype have some properties which are specific to the type of object we are copying. If we are creating and array like anarray
then prototype of Array.constructor
function will be attached to anarray
. If we try to get the prototype of Array which will refer to its constructors prototype will be exact same copy of the prototype of anarray.constructor
. Lets understand this with code.
Object.getPrototypeOf(Array) === Object.getPrototypeOf(anarray.constructor); // this statement will return true
Lets see what does the prototype of an array looks like.
Object.getPrototypeOf(anarray); // this will output the prototype of an array and all its methods we normally use
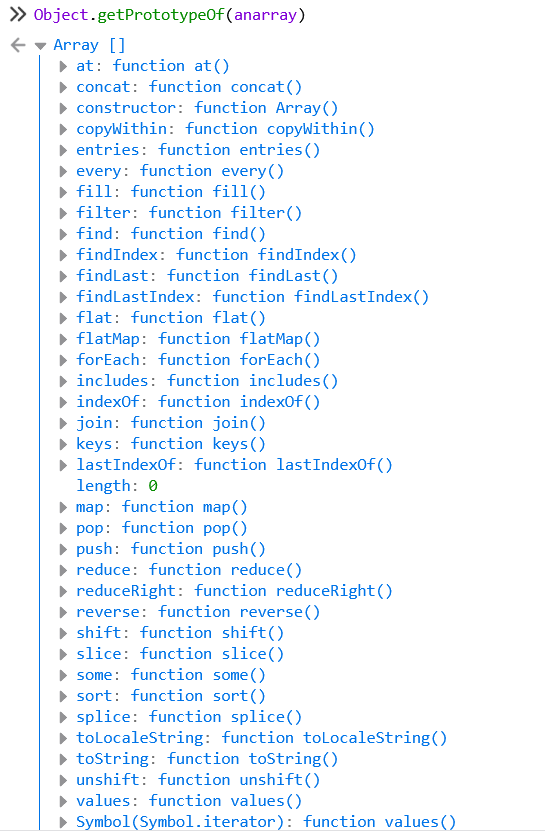
Similar to above array example, we can create string, variables, objects and class and can see their prototypes with Object.getPrototypeOf()
function.
What is __proto__
in JavaScript?
__proto__
is reference to prototype
( or it points towards prototype
), if we want to access prototype, we use __proto__
.
But __proto__ is now depreciated.
Deprecated: This feature is no longer recommended. Though some browsers might still support it, it may have already been removed from the relevant web standards, may be in the process of being dropped, or may only be kept for compatibility purposes. Avoid using it, and update existing code if possible; see the compatibility table at the bottom of this page to guide your decision. Be aware that this feature may cease to work at any time.
https://developer.mozilla.org/
What is __proto__
alternative in JavaScript?
Note: The use of
https://developer.mozilla.org/__proto__
is controversial and discouraged. Its existence and exact behavior have only been standardized as a legacy feature to ensure web compatibility, while it presents several security issues and footguns. For better support, preferObject.getPrototypeOf()
/Reflect.getPrototypeOf()
andObject.setPrototypeOf()
/Reflect.setPrototypeOf()
instead.
Before
a = element.__proto__
b = element.__ptoto__.__proto__
After
a = Object.getPrototypeOf(element)
b = Object.getPrototypeOf(a)
What is prototype chaining?
Prototype object has a prototype of its own, and so on until an object is reached with null as its prototype, this is called prototype chaining.
What is prototypal inheritance?
We can inherit the properties of one object in other object by assigning other object as a prototype of first object. Now if we will try to access the properties first object, JavaScript will search for that property in first object and if not found then it will look for that in its prototypes until that property is found or null values of prototype is received. This behavior is also called prototype chaining which we discussed above.
Example:
let object1 = {name:"Sahil"}
let Object2 = {lastname:"Ahlawat"}
// now lets inherit object2 in object 1 with prototypal inheritance
Object.setPrototypeOf(object1,object2);
object1.name; // this will give Sahil
object.lastname; // this will give Ahlawat
How to extend a prototype?
There are many usefull properties that are provided by prototypes by default like array sort, array length, different loops and many more. What if we want to create our own such properties?
Yes we can extend prototypes to make our life easier.
Lets try to a custom function for Array so all new arrays that we create in our code can use that function.
Array.prototype.customfunction = function(){return "Hello from Sahil";}
We have extended the prototype of Array object in JavaScript, now if we will create any child object, we will be able to access this custom function with that.
let arr = [];
console.log(arr.customfunction()); // this will print Hello from Sahil in console
Conclusion
Prototypes are soul of JavaScript as we know it. We can understand them, we can extend them and can use them as we want.
A freelance web developer with a decade of experience in creating high-quality, scalable web solutions. His expertise spans PHP, WordPress, Node.js, MySQL, MongoDB, and e-commerce development, ensuring a versatile approach to each project. Aadi’s commitment to client satisfaction is evident in his track record of over 200 successful projects, marked by innovation, efficiency, and a customer-centric philosophy.
As a professional who values collaboration and open communication, Aadi has built a reputation for delivering projects that exceed expectations while adhering to time and budget constraints. His proactive and problem-solving mindset makes him an ideal partner for anyone looking to navigate the digital landscape with a reliable and skilled developer.